The Lightweight Python Web Framework Flask for Rapid Development
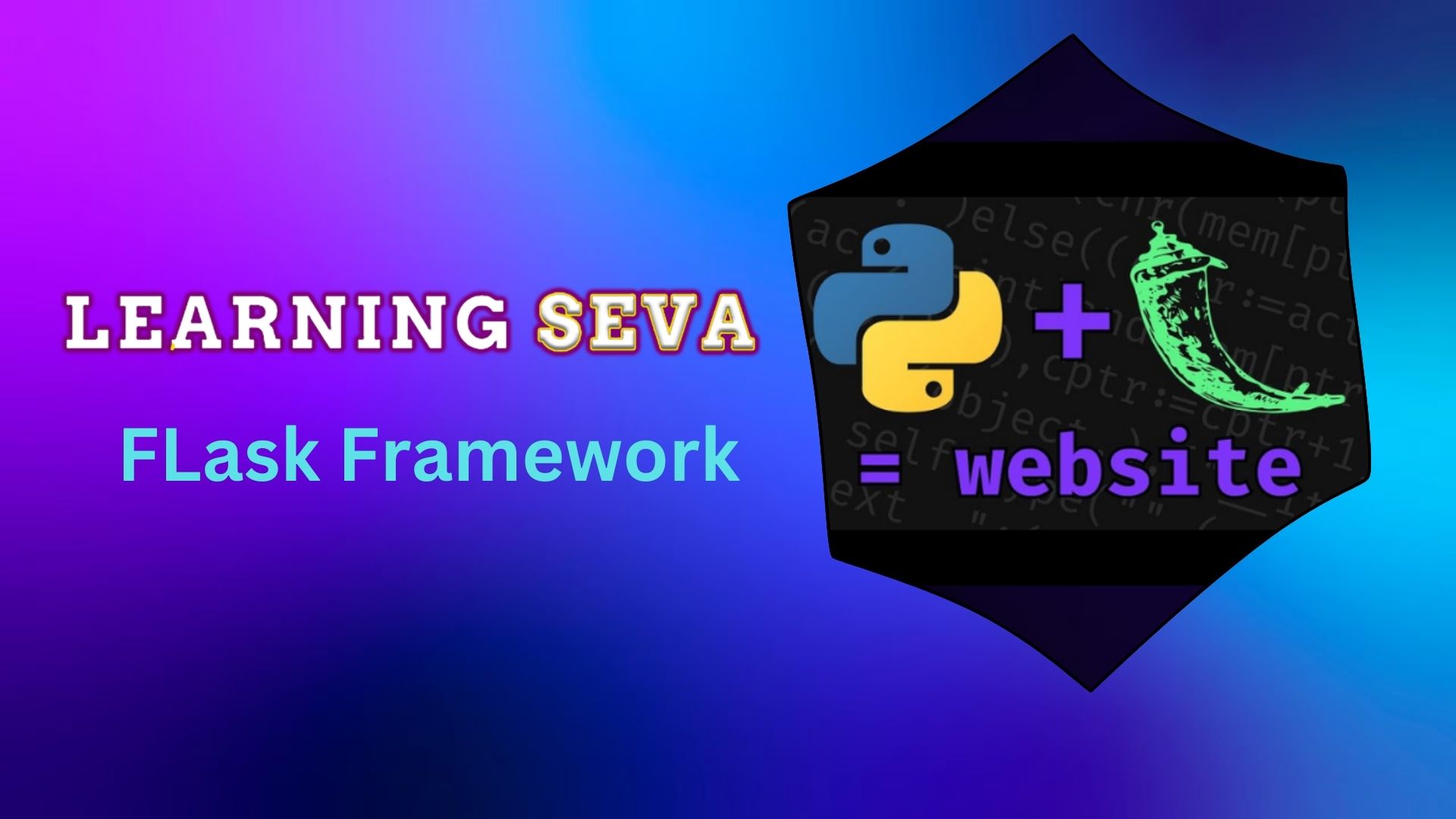
About Course
The Flask course is designed to provide a comprehensive understanding of Flask, a lightweight WSGI web application framework written in Python. This course is suitable for beginners and experienced developers looking to build scalable web applications quickly and efficiently.Participants will learn how to create dynamic web applications using Flask’s core features, such as routing, templates, and database integration. The course emphasizes practical applications, allowing learners to implement their knowledge through hands-on projects.
Description
This course covers a wide range of topics in Flask development, from basic concepts to advanced features. Students will engage in practical exercises and projects that reinforce learning and facilitate real-world application.Key topics include:
- Introduction to Flask: Understanding the framework’s history, installation, and setup.
- Routing: Defining URL routes and handling requests.
- Templates with Jinja2: Using the Jinja2 template engine to render dynamic HTML pages.
- Forms Handling: Managing user input through forms and implementing validation.
- Database Integration: Connecting Flask applications to databases using SQLAlchemy or other ORMs.
- Building RESTful APIs: Creating APIs for web services using Flask’s capabilities.
- User Authentication: Implementing user login systems and managing sessions.
What Will I Learn?
- Good Work: Master the fundamentals of C programming for effective software development.
- Stage Fear: Gain confidence in tackling complex challenges.
- Talk: Develop skills to communicate concepts clearly.
- 5 PLUS Year Industry Experience: Learn from industry experts with real-world applications.
FAQs
Flask is a lightweight WSGI web application framework written in Python. It is designed to make getting started quick and easy while allowing for scalability to complex applications. Flask is classified as a microframework because it does not require particular tools or libraries.
Flask was developed by Armin Ronacher as part of the Pocoo project, which is a collection of Python libraries.
Key features include:
- Simple and easy to use
- Lightweight and modular
- Built-in development server and debugger
- Integrated support for unit testing
- URL routing and request handling
- Jinja2 templating engine for rendering HTML
You can install Flask using pip, Python’s package manager. Run the following command:
pip install Flask
You can create a new Flask application by creating a Python file (e.g., app.py
) and adding the following code:
from flask import Flask app = Flask(__name__) @app.route(‘/’) def hello(): return ‘Hello, World!’ if __name__ == ‘__main__’: app.run(debug=True)
To run your Flask application, execute the following command in your terminal:
python app.py
This will start the development server, typically accessible at http://127.0.0.1:5000/
.
URL routing in Flask allows you to map URLs to specific functions (views) in your application using decorators like @app.route()
.
Flask uses Jinja2 as its template engine. You can render templates using the render_template()
function:
You can handle forms in Flask using the request
object to access form data submitted via POST requests:
Flask does not come with built-in database support but can be integrated with SQLAlchemy or other ORMs by installing additional packages.
Flask provides several security features including protection against cross-site scripting (XSS), cross-site request forgery (CSRF), and secure cookies for session management.
Flask provides testing capabilities through its built-in test client, allowing you to simulate requests to your application:
import unittest class MyTestCase(unittest.TestCase): def setUp(self): self.app = app.test_client() def test_hello(self): response = self.app.get(‘/’) self.assertEqual(response.data, b’Hello, World!’)
Flask applications can be deployed on various platforms such as Heroku, AWS, or DigitalOcean using WSGI servers like Gunicorn or uWSGI.
Yes! Flask supports many extensions that provide additional functionality such as authentication, database integration, form validation, and more.
Yes! The Flask community is active with numerous forums, mailing lists, and resources available for developers seeking help or sharing knowledge.
Resources include:
- Official documentation at flask.palletsprojects.com
- Online courses on platforms like Uptaught, and Coursera
- Tutorials on websites like Real Python and W3Schools
Common issues include understanding routing, managing dependencies, configuring settings properly, and debugging errors.
Yes! You can create RESTful APIs using Flask by defining routes that respond to HTTP methods (GET, POST, PUT, DELETE) and returning JSON responses.
Middleware refers to functions that process requests globally before they reach views or after they leave views, allowing you to add functionality such as logging or authentication.
Signals allow certain senders to notify receivers when certain actions occur within your application, enabling decoupled components.
A course by
Material Includes
- Material 1
- Material 2
- Material 3
Requirements
- Laptop
- Good Internet Connection
- Graduated
- 1 Year Industry Experience