Complete C# Certification Course: From Basics to Expert-Level Coding
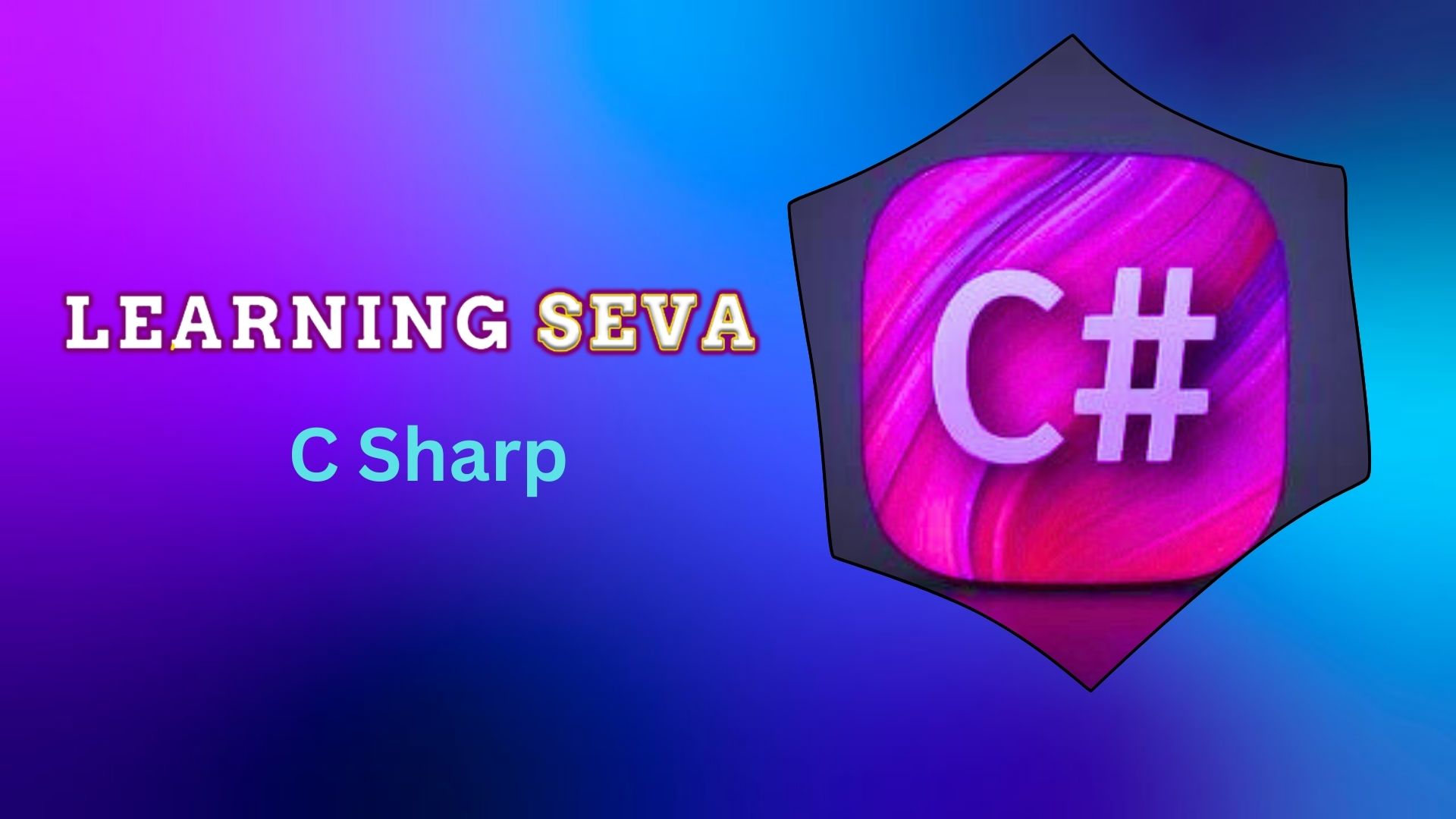
About Course
The C# course is designed to provide a comprehensive understanding of C#, a modern, object-oriented programming language developed by Microsoft. This course is suitable for beginners as well as experienced developers looking to enhance their skills in building applications for the .NET framework.Participants will learn the fundamentals of C#, including syntax, data types, control structures, and object-oriented programming principles. The course emphasizes hands-on experience, allowing learners to implement their knowledge through practical projects and real-world scenarios.
Description
This course covers a wide range of topics related to C#, from foundational concepts to advanced features. Students will engage in practical exercises that reinforce learning and facilitate real-world application.Key topics include:
- Introduction to C#: Understanding the history of C# and its role within the .NET ecosystem.
- Basic Syntax and Data Types: Learning about variables, data types, operators, and control structures.
- Object-Oriented Programming (OOP): Exploring concepts such as classes, objects, inheritance, polymorphism, and encapsulation.
- Exception Handling: Implementing error handling using try-catch blocks.
- LINQ (Language Integrated Query): Using LINQ for data manipulation and querying collections.
- Asynchronous Programming: Understanding async and await for managing asynchronous operations.
- Building Applications: Creating console applications and Windows Forms applications using C#.
What Will I Learn?
- Good Work: Master the fundamentals of C programming for effective software development.
- Stage Fear: Gain confidence in tackling complex challenges.
- Talk: Develop skills to communicate concepts clearly.
- 5 PLUS Year Industry Experience: Learn from industry experts with real-world applications.
FAQs
C# (pronounced “C-sharp”) is a modern, object-oriented programming language developed by Microsoft as part of its .NET initiative. It is designed for building a wide range of applications, from web and mobile apps to enterprise software and games.
Key features include:
- Object-Oriented: Supports encapsulation, inheritance, and polymorphism.
- Type Safety: Provides strong type checking at compile time.
- Automatic Memory Management: Uses garbage collection to manage memory allocation and deallocation.
- Rich Standard Library: Offers a comprehensive set of libraries for various functionalities.
- Cross-Platform Development: With .NET Core and .NET 5/6, C# applications can run on Windows, macOS, and Linux.
You can use various Integrated Development Environments (IDEs) such as:
- Visual Studio: A powerful IDE with extensive features for C# development.
- Visual Studio Code: A lightweight code editor with support for C# through extensions.
- Rider: A cross-platform IDE from JetBrains specifically designed for .NET development.
A class can be defined using the class
keyword:
C# supports several data types including:
- Value Types: int, float, double, char, bool, etc.
- Reference Types: string, arrays, classes, interfaces, etc.
Control structures such as if
, switch
, for
, and while
are used to control the flow of execution:
C# uses try
, catch
, and finally
blocks to handle exceptions:
try { // Code that may throw an exception } catch (Exception ex) { // Handle the exception } finally { // Code that runs regardless of whether an exception occurred }
The four main principles of OOP in C# are:
- Encapsulation: Bundling data and methods that operate on the data within one unit (class).
- Inheritance: Creating new classes based on existing ones to promote code reuse.
- Polymorphism: Allowing methods to perform differently based on the object calling them.
- Abstraction: Hiding complex implementation details and exposing only necessary parts.
LINQ (Language Integrated Query) is a feature that allows you to query collections of data using a syntax similar to SQL directly within C#. It provides a more readable and concise way to work with data sources.
C# supports asynchronous programming using the async
and await
keywords, allowing you to write non-blocking code that can handle long-running tasks without freezing the application:
public async Task<string> GetDataAsync() { using (HttpClient client = new HttpClient()) { var response = await client.GetStringAsync(“https://api.example.com/data”); return response; } }
Common frameworks include:
- ASP.NET Core: For building web applications and APIs.
- Entity Framework Core: An ORM for database access.
- Xamarin: For building mobile applications using C#.
- Blazor: For building interactive web UIs using C# instead of JavaScript.
Yes! The C# community is active with forums like Stack Overflow, Microsoft Q&A, GitHub repositories, and user groups where developers can seek help or share knowledge.
Resources include:
- Official documentation at docs.microsoft.com
Common challenges include understanding object-oriented concepts, managing memory effectively, debugging code efficiently, and navigating the .NET ecosystem.
A course by
Material Includes
- Material 1
- Material 2
- Material 3
Requirements
- Laptop
- Good Internet Connection
- Graduated
- 1 Year Industry Experience